- JiBX is fast!
- JiBX has a small footprint (it will add only 60KB to your .apk)
- JiBX uses the Android XMLPull parser by default
- It's easy to use!
We all appreciate the advantages of being able to use java classes to do data manipulation.
person.setFirstName("Don");
is much easier then messy DOM manipulation: document.getElementsByTagName("first").item(0).appendChild(document.createTextNode("Don"));
Let's create a sample project.
First, create java bindings for your XML schema using JiBX, or select a pre-build schema library.
For this example, we'll use a pre-built binding from the JiBX schema library. Our sample xsd file is an XML definition which includes a person's first and last name.
Click here to see our sample schema in the JiBX library. You may want to take a look at the schema and the JiBX generated java source code.
Download the java jar file by right-clicking this
link and select 'save as'.
Next, download the jibx runtime jar file by right-clicking this link and select 'save as'. We'll need these jars shortly.
Now we're ready to create our Android application. First you need to install eclipse and the Android SDK.
From Eclipse, select New -> Project -> Android Project.
I'm calling this project 'jibxapp'.
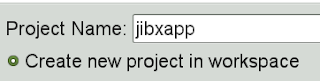
On the next screen, select your target api.

Finally, enter your java package name.
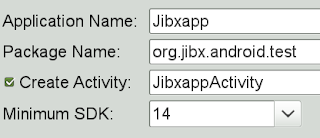
You project should appear in your workspace.
Navigate to jibxapp -> res -> layout and double-click main.xml to bring up the form editor.
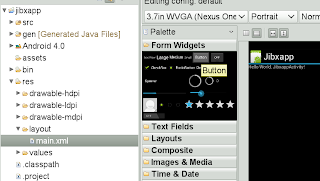
Add a button, two medium static text fields, two data text boxes, and a multi-line text box so your screen looks something like this:
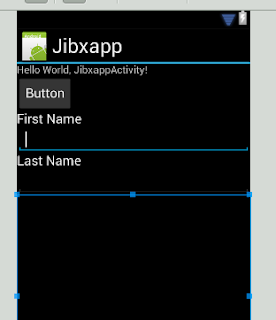
You may want to test your Android emulator here by right clicking the project name and selecting 'Debug as' -> 'Android Application'.
To add your java binding and the JiBX runtime, right-click your project name and select properties. Click 'java build path' -> Libraries -> 'Add external jars' and add the two jars that we downloaded earlier.
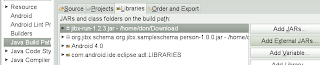
Navigate and open your Android source code file.
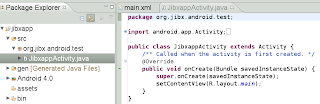
Add this test code to your java source file:
package org.jibx.android.test;
import java.io.ByteArrayOutputStream;
import java.io.StringReader;
import java.io.UnsupportedEncodingException;
import org.jibx.runtime.BindingDirectory;
import org.jibx.runtime.IBindingFactory;
import org.jibx.runtime.IMarshallingContext;
import org.jibx.runtime.IUnmarshallingContext;
import org.jibx.runtime.JiBXException;
import org.jibx.schema.org.jibx.sampleschema.person.Person;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class JibxappActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
mPickDate = (Button) findViewById(R.id.button1);
mFirstName = (EditText) findViewById(R.id.editText1);
mLastName = (EditText) findViewById(R.id.editText2);
mTextBox = (EditText) findViewById(R.id.editText3);
// add a click listener to the button
mPickDate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(android.view.View v) {
doJibxTest();
}
});
}
Button mPickDate = null;
EditText mFirstName = null;
EditText mLastName = null;
EditText mTextBox = null;
/**
* Add the test table records.
*/
public void doJibxTest() {
Person person = new Person();
person.setFirstName(mFirstName.getText().toString());
person.setLastName(mLastName.getText().toString());
String xml = marshalMessage(person);
mTextBox.setText(xml);
Person personOut = (Person) unmarshalMessage(xml);
mPickDate.setText(personOut.getFirstName() + ' ' + personOut.getLastName());
}
/**
* Marshal this message to xml .
*
* @param message
* @param system
* @return
*/
public final static String STRING_ENCODING = "UTF8";
public final static String URL_ENCODING = "UTF-8";
String bindingName = "binding";
public String marshalMessage(Object message) {
try {
IBindingFactory jc = BindingDirectory.getFactory(bindingName, Person.class);
IMarshallingContext marshaller = jc.createMarshallingContext();
ByteArrayOutputStream out = new ByteArrayOutputStream();
marshaller.marshalDocument(message, URL_ENCODING, null, out);
return out.toString(STRING_ENCODING);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
} catch (JiBXException e) {
e.printStackTrace();
}
return null;
}
/**
* Unmarshal this xml Message to an object.
* @param xml
* @param system
* @return
*/
public Object unmarshalMessage(String xml) {
try {
IBindingFactory jc = BindingDirectory.getFactory(bindingName, Person.class);
IUnmarshallingContext unmarshaller = jc.createUnmarshallingContext();
return unmarshaller.unmarshalDocument(new StringReader(xml), bindingName);
} catch (JiBXException e) {
e.printStackTrace();
}
return null;
}
}
This program takes the First and Last Name entered, plugs them into a java data object, marshals the object to xml, and unmarshals the xml back to a data object and extracts the data from it. I made this program very simple, of course you would not do this kind of processing in the main thread.
Run your application by right clicking the project name and selecting 'Debug as' -> 'Android Application'. Enter your first and last name and click the button on the top.
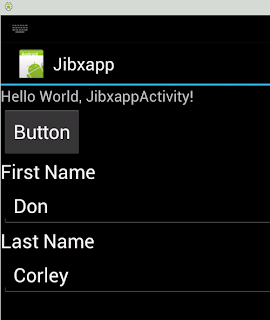
Voilà!
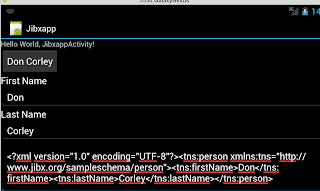
53 comments:
You can find this project in the JiBX source repository at:
https://github.com/jibx/schema-library/tree/master/schema-utilities/examples/android/jibxapp
In Android Development,it doesn't perform bytecodes. Android's java runtime uses a register based virtual machine that completes .dex information instead of class information. Theoretically you could generate those instead of java bytecode.
First, I think the author did a great job to make JiBX work on Android, however, JiBX was originally designed for desktop and server side development, hence it's too heavy-weight for resource limited mobile device like Android. I would recommend a light-weight xml binding framework tailed for Android platfrom, the library is called Nano, the jar is less than 70K, performance comparable to Android native parser like SAX and Xml Pull, it supports both XML and Json binding, it uses annotation driven binding, similar to JAXB, also it provides a binding compiler to auto-generate bindable class from xml schema, you may have a try if you are interested in it.
https://github.com/bulldog2011/nano
William,
Actually JiBX was designed to be small and fast. It uses the xmlpull parser which is built into Android. It is perfect for the Android platform.
Thanks for the detailed article!
After failing with some Java XML projects, JiBX did the work.
Thank you.
Just as a side note, for anyone who with to have a generic (un)marshal methods:
public static String marshalMessage(T message, Context ctx) {
try {
IBindingFactory jc = BindingDirectory.getFactory(bindingName, message.getClass());
IMarshallingContext marshaller = jc.createMarshallingContext();
ByteArrayOutputStream out = new ByteArrayOutputStream();
marshaller.marshalDocument(message, URL_ENCODING, null, out);
return out.toString(STRING_ENCODING);
}catch (Exception e){
e.printStackTrace();
}
return null;
}
public static T unmarshalMessage(String xml, Class T) {
try {
IBindingFactory jc = BindingDirectory.getFactory(bindingName, T);
IUnmarshallingContext unmarshaller = jc.createUnmarshallingContext();
return (T) unmarshaller.unmarshalDocument(new StringReader(xml), bindingName);
} catch (JiBXException e) {
e.printStackTrace();
}
return null;
}
That’s a great article. But there is more to do if you learn Android. Just try visiting our page!
Android Training Chennai
That is a brilliant article on dot net training in Chennai that I was searching for. Helps us a lot in referring at our dot net training institute in Chennai. Thanks a lot. Keep writing more on dot net course in Chennai, would love to follow your posts and refer to others in dot net training institutes in Chennai.
Quite Interesting post!!! Thanks for posting such a useful post. I wish to read your upcoming post to enhance my skill set, keep blogging.
Regards,
CCNA Training in Chennai | CCNA Training Institute in Chennai | Best CCNA Training in Chennai
Nice tutorial on android technology hats-off to your effort. Your article explained the potential of android technology in coming years. Android Training in Chennai|iOS Training in Chennai |Android training
Fantastic post...Android Training in Chennai | Best Android Training in Chennai | Android Training
Excellent post.You gave a detailed explanation.
Thanks
DOT NET Course Chennai
| DOT NET Training Institute in Chennai
Hadoop is especially used for Big Data maintenance. It uses Hadoop distributed file system . Its operating system is in cross platform. Its framework mostly written in java programming language. The other languages which are used by hadoop framework are c, c++ (c with classes) and sometimes in shell scripting.Thank you..!!
regards,
Hadoop Training in Chennai | hadoop training in adyar
Thanks Admin for sharing such a useful post, I hope it’s useful to many individuals for whose looking this precious information to developing their skill.
Regards,
Android Training in Chennai|Android Course in Chennai|Android Training Chennai|iOS Training|iOS Course in Chennai
Mettur dam updates Mettur Dam
Mettur dam updates Mettur
Hi I am from Chennai. Thanks for sharing the informative post about Android technology. It’s really useful for me to know more about this technology. Recently I did Android Training in Chennai.
Android Training in Chennai
I love reading through your blog, I wanted to leave a little comment to support you and wish you a good continuation. Wish you best of luck for all your best efforts. I want to share some information, we provide outsource data entry services in all over the world.
Back Office Services
I wondered upon your blog and wanted to say that I have really enjoyed reading your blog posts. Any way I’ll be subscribing to your feed and I hope you post again soon.
mobile website builder
Interesting post! This is really helpful for me. I like it! Thanks for sharing!
Mobile application developers in Chennai | PHP developers Chennai
Thanks a lot very much for the high quality and results-oriented help.
I won’t think twice to endorse your blog post to anybody who wants
and needs support about this area.
java training in chennai
java training in bangalore
I simply wanted to write down a quick word to say thanks to you for those wonderful tips and hints you are showing on this site.
big-data-hadoop-training-institute-in-bangalore
Best Php Training Institute in Delhi
Best Java Training Institute in delhi
linux Training center in delhi
Web Designing Training center in delhi
Oracle Training Institute in delhi
blue prism Training Institute in delhi
Automation Anywhere Training center In delhi
rpa Training Institute in delhi
hadoop Training center in delhi
guys if you make your carrier and do what you want to do in your life so webtrackker is the best option to take your carrier make large
You're the best particularly for the high caliber and results-situated help. I won't reconsider to embrace your blog entry to anyone who needs also, needs bolster about this zone.
planet-php
nice post if anyone searching best training institute then visit android summer training
java summer training
Your post is nice if anyone searching for the training in java can visit Java Training in Tambaram
How can I doto use my own java class instead the options provided by jibx?
I reading your post from the beginning, it was so interesting to read & I feel thanks to you for posting such a good blog, keep updates regularly..
Java Training in Chennai | Java Training Institute in Chennai
You're really great especially for the high gauge and results-arranged help. I won't reevaluate to grasp your blog passage to any individual who needs additionally, needs support about this zone.
Education | Article Submission sites | Technology
Best institute for 3d Animation and Multimedia Course training Classes
Best institute for 3d Animation and Multimedia
Best institute for 3d Animation Course training Classes in Noida- webtrackker Is providing the 3d Animation and Multimedia training in noida with 100% placement supports. for more call - 8802820025.
3D Animation Training in Noida
Company Address:
Webtrackker Technology
C- 67, Sector- 63, Noida
Phone: 01204330760, 8802820025
Email: info@webtrackker.com
Website: http://webtrackker.com/Best-institute-3dAnimation-Multimedia-Course-training-Classes-in-Noida.php
Very interesting content which helps me to get the indepth knowledge about the technology. To know more details about the course visit this website.
iOS Training in Chennai
Big Data Training in Chennai
Hadoop Training in Chennai
Android Training in Chennai
Selenium Training in Chennai
Digital Marketing Training in Chennai
Well written post with worthy information. It will definitely be helpful for all. Do post more like this.
Blue Prism Training in Chennai
Blue Prism Training in Anna Nagar
RPA Training in Chennai
RPA course in Chennai
UiPath Training in Chennai
RPA Training in Velachery
UiPath Training in Chennai
Blue Prism Training in Chennai
Thank you very much for the details you shared. Kindly try to implement a kind of information through it and keep writing such wonderful things.
Job Openings in Chennai
job vacancy in chennai
Pega Training in Chennai
Oracle Training in Chennai
Embedded System Course Chennai
Linux Training in Chennai
Unix Training in Chennai
Power BI Training in Chennai
Oracle DBA Training in Chennai
Tableau Training in Chennai
Spark Training in Chennai
Great Articles!!!Thanks for sharing with us
Java training in chennai | Java training in annanagar | Java training in omr | Java training in porur | Java training in tambaram | Java training in velachery
Thanks a lot very much for the high quality and results-oriented help. I won’t think twice to endorse your blog post to anybody who wants and needs support
java training in chennai
java training in omr
aws training in chennai
aws training in omr
python training in chennai
python training in omr
selenium training in chennai
selenium training in omr
It's a wonderful post and very helpful, thanks for all this information about Java. You are including better information regarding this topic in an effective way. Thank you so much.
hardware and networking training in chennai
hardware and networking training in tambaram
xamarin training in chennai
xamarin training in tambaram
ios training in chennai
ios training in tambaram
iot training in chennai
iot training in tambaram
Wow its very useful and you explained detailed,
Thanks to share with us,
sap training in chennai
sap training in porur
azure training in chennai
azure training in porur
cyber security course in chennai
cyber security course in porur
ethical hacking course in chennai
ethical hacking course in porur
Detailed article with clear explanation.
sap training in chennai
sap training in annanagar
azure training in chennai
azure training in annanagar
cyber security course in chennai
cyber security course in annanagar
ethical hacking course in chennai
ethical hacking course in annanagar
"It was an informative post indeed. Now It's the time to make the switch to solar power,
contact us(National Solar Company) today to learn more about how solar power works.
solar panels
solar inverter
solar batteries
solar panels adelaide
best solar panels
solar power
battery storage solar
battery charger solar
solar regulators
solar charge controllers
solar battery storage
instyle solar
solar panels melbourne
solar panels for sale
solar battery charger
solar panels cost
buy solar panels"
Quite Interesting post!!! Thanks for posting such a useful post.
acte chennai
acte complaints
acte reviews
acte trainer complaints
acte trainer reviews
acte velachery reviews complaints
acte tambaram reviews complaints
acte anna nagar reviews complaints
acte porur reviews complaints
acte omr reviews complaints
Hi,here im sharing my own experience how i earned money using online.go throught the given website to know more details.
Easy way to earn money online tips and tricks
Earn money online tips and tricks
Earn money online without investments
Affliate Marketing websites to earn money
Very nice information. Thank you so much to share this with us.
Java Training in Chennai
Java Training in Velachery
Java Training inTambaram
Java Training in Porur
Java Training in Omr
Java Training in Annanagar
this coding is very use full to me and xml in java coding is clearly explained, thank u so much for this amzing blog and keep posting
Software Testing Training in Chennai
Software Testing Training in Velachery
Software Testing Training in Tambaram
Software Testing Training in Porur
Software Testing Training in Omr
Software Testing Training in Annanagar
Thanks for your article on Android technology. Android is an open source platform that allows developers to create stunning website
Digital Marketing Training in Chennai
Digital Marketing Training in Velachery
Digital Marketing Training in Tambaram
Digital Marketing Training in Porur
Digital Marketing Training in Omr
Digital MarketingTraining in Annanagar
Hi, Great.. Tutorial is just awesome..It is really helpful for a newbie like me.. I am a regular follower of your blog. Really very informative post you shared here. Kindly keep blogging.salesforce training in chennai
software testing training in chennai
robotic process automation rpa training in chennai
blockchain training in chennai
devops training in chennai
Great! This article is packed full of constructive information. The valuable points made here are apparent, brief, clear and poignant. I am sincerely fond of your writing style.
SAP training in Mumbai
SAP course in Mumbai
"Bruce Caplan is a Licensed Insolvency Trustee with over 25 years of experience helping people and companies
solve their debt problems.
Winnipeg Consumer Proposal
Consumer Proposal Winnipeg
Bankruptcy Winnipeg
Insolvency Winnipeg
Winnipeg Bankruptcy
consumer proposal
Winnipeg Insolvency
Winnipeg Insolvency Trustee
Insolvency Trustee Winnipeg
Bankruptcy
Winnipeg Debt Consolidation
Credit Counselling Winnipeg
Debt Consolidation Winnipeg
Debt Consolidation loan"
Your post is really good. It is really helpful for me to improve my knowledge in the right way..
I got more useful information from this thanks for share this blog
scope of automation testing
scope of data science
how to improve english
common grammar mistakes
nodejs interview questions
pega interview questions
Communication is a two-way process. If done properly, it gives an excellent result. Thus opting for the best Integrated Marketing Communication Course on Talentedge is wise. To know more visit:
Visit Bharat Go Digital Academy to learn the digital marketing skills in India.
Quick Heal Total Security License Key is an antivirus created by Quick Heal Technologies. It is a lightweight cloud-based protection software. Quick Heal Product Installer
Design new sites visually with the popular Site Designer app or edit the code for existing projects manually Coffee Web Form Builder
Jumma Mubarak! Keep me in your dua, and I shall remember you in mine! May this Jumma bear divine blessings for us! Wishing you a graceful Jumma! Jummah Mubarak Quote
Click through our link and create your Spin Casino account to 바카라사이트 claim our exclusive sign up bonus. Use your particulars to log in or create a fresh account for the mobile gaming world to suit the palm of your hand. The Spin Mobile Casino iOS app is necessary thing} that opens the iPhone chamber of delights for the Kiwi faithful.
Post a Comment